Week 1
1) What best defines a "programming language"?
- It allows us to control a computer.
- It allows us to make a calculation.
- It allows to execute a program.
- It allows us to express an algorithm.
2) Select the valid identifiers from the following list. State why each of the others is not valid.
- 123
- $50
- 50dollars
- abs
- hunter
- @60cents
- a_b
- #define
3) We learned about basic programs such as Hello World!
How can we modify this to print a whole message?
Let's print two lines:
- First: Hello, my name is [YOUR NAME HERE].
- Second: How are you?
4) Write a Java program that creates TWO integer variables to the values of your choice (e.g. 4 and 5, 12 and 10, etc.) and computes and prints the following:
- the sum of the two numbers
- the difference between the two numbers
- the product of the two numbers
- the quotient of the two numbers
5) Answer the following:
- What is the result of the following Java expression: 5 + 6 * 3 ?
- How does the order of operations affect the evaluation of the following expression: 4 + 5 * 2 - 3 ?
- Evaluate the following Java expression: 10 / 2 + 3 * 5 - 4
-
What is the output of the following code snippet?
int a = 2 + 3 * 4 - 1; System.out.println("Result: " + a);
- Rewrite the following expression using parentheses to explicitly define the order of operations: 8 - 2 * 4 / 2 + 3
-
What is the value of the variable 'b' after executing the following
code snippet?
int b = 10; b += 2 * 3 - 1;
6) Trace the following program:
public static void main(String [] args) {
int a, b, c, d, e, f, g;
a = 10;
b = 27;
System.out.println("a is " + a + ", b is " + b);
c = a + b;
System.out.println("a+b is " + c);
d = a - b;
System.out.println("a-b is " + d);
e = a+b*3;
System.out.println("a+b*3 is " + e);
f = b / 2;
System.out.println("b/2 is " + f);
g = b % 10;
System.out.println("b%10 is " + g);
}
7) Write a Java program to print the area and perimeter of a rectangle.
- initialize two integer variables:
- one for length
- one for width
- compute the formula for area: a = wl
- compute the formula for perimeter: p = 2(l+w)
- print and label the results
8) Write a Java program to convert temperature from Fahrenheit to Celsius degrees.
9) What will be printed by the following program?
public static void main(String [] args) {
int w, x;
double y;
w = -9;
x = Math.abs(w + 5);
y = Math.sqrt(x);
System.out.printf("%d %d %.2f%n", w,x,y);
}
10) Assuming that the variable x has already been declared and assigned a value, is the following assignment legal? If not why?
11) Write a Java program that initializes the radius of a circle and computes and prints the circle's area and circumference (perimeter). (Limit each result to a precision of 3 decimal places)
- Formula for area: πr^2
- Formula for circumference (perimeter): 2πr
12) Write a Java expression that would compute the following:
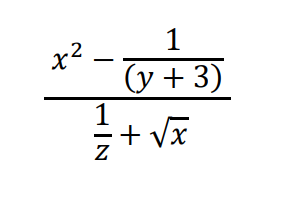
Review
a) Write a statement that reads an integer value from standard input into val. Assume that val has already been declared as an int variable. Assume also that stdin is a variable that references a Scanner object associated with standard input.
b) Assume the availability of a class named Arithmetic that provides a static method,
add, that accepts two int arguments and returns their sum.
Two int variables, euroSales and asiaSales, have already been declared
and initialized. Another int variable, eurasiaSales, has already been declared.
Write a statement that calls add to compute the sum of euroSales and asiaSales
and that stores this value in eurasiaSales.
c) Given the variable pricePerCase, write an expression corresponding to the price of a dozen cases.
e) Write a Java expression that would compute the following:
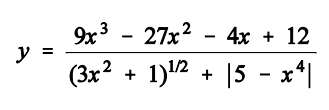
1) Write a Java program that computes the cost of traveling between two cities.
Prompt for and read in the name of each city (two separate prompts),
the distance (in miles) between the two cities, and the cost of travel per mile.
Run the program with the following data:
- Cincinnati, Columbus, 115 and 0.50
Your output should look like:
- The cost of traveling from Cincinnati to Columbus is $57.50
Lab 1: One Guess Game
The goal of this exercise is to program a Guess My Number game. When it's finished, it should work like this:
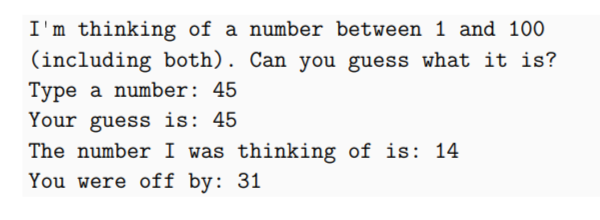
- First, use Math.random() to generate a number between 1-100 (inclusive)
- Prompt the user to enter their guess and use a Scanner to read in user input
- Display the user input and the random number
- Finally, compute and display the difference between the user's guess and the number that was generated (hint: use absolute value!)
- Write all output (not prompts!) to a plain text file called: "oneGuessGame_Results.txt"
Submit both the .java and .txt file to EC Lab Submissions under Class Labs on Blackboard before next class. (This lab will count as part of your CodeLab grade)
2) Three business partners are forming a company whose name will be of the form "Name1, Name2, and Name3".
However, they can't agree whose name should be first, second or last.
Help them out by writing code that
reads in their
three names and prints each possible combination exactly once, on a line by itself (that is, each possible
combination is
terminated with a newline character).
Assume that name1, name2, and name3 have already been declared
and use them in your code.
Assume also that stdin is a variable that references a Scanner object associated with standard input.
For example, if your code read
in Larry, Curly, and Moe it would print out "Larry, Curly, and Moe", "Curly, Larry, and Moe", etc., each on a
separate line.
(CodeLab: Chapter 3: Input and output > 3.2: The Scanner class > #20966)
3) Write a Java program that converts a total number of seconds to hours, minutes, and seconds.
It should:
- prompt the user for input
- read an integer from the keyboard
- calculate the result
- and use printf to display the output
For example:
"5000 seconds = 1 hours, 23 minutes,
and 20 seconds".
(Hint: Use the modulus operator)
4) Write a Java program to prompt the user to enter their first and last name, then generate a random integer
between 1000 - 9999 (inclusive)
and write it to a file as follows:
'[last name]_[first_name]_Info.txt'
Then read from the newly created file and print out the contents (it should be the same as you just entered,
plus the ID number)
Review
a) Two variables, num and cost have been declared and given values: num is an integer and cost is
a double.
Write a single statement that outputs num and cost to standard output. Print both values (num first, then
cost),
separated by a space on a single line that is terminated with a newline character. Do not output any thing
else.
(CodeLab: Chapter 2: Variables and operators > 2.4: Printing variables > #20979)
1) Write a Java program that prompts the user and reads in an integer and check whether it is even or odd.
Input Example:
- Input a number: 7
Expected Output:
- This number is odd.
2) Write a Java statement to accomplish each of the following:
- set q equal to the square root of 15 if v is negative
- set h to 3 if count is a multiple of 10
- use the ternary operator to set i equal to 7 if x is not negative or 8 otherwise
3) Write a Java program that prompts for three numbers from the user and prints out the largest number of the three.
Input Example:
- Enter the first number: 25
- Enter the second number: 78
- Enter the third number: 87
Expected Output:
- The largest number is: 87
4) Write an if/else statement that compares the double variable pH with 7.0 and makes the following assignments to the int variables neutral, base, and acid:
- 0,0,1 if pH is less than 7
- 0,1,0 if pH is greater than 7
- 1,0,0 if pH is equal to 7
5) Write a Java program to find the number of days in a month from a particular year.
First, we need to prompt the user for the month and the year:
Input Example:
- Input a month number: 2
- Input a year: 2016
Remember:
- January: 31 days
- February: 28 days
(29 days in a leap year) - March: 31 days
- April: 30 days
- May: 31 days
- June: 30 days
- July: 31 days
- August: 31 days
- September: 30 days
- October: 31 days
- November: 30 days
- December: 31 days
Every year that is exactly divisible by four is a leap year, except for years that are exactly divisible by 100, but these centurial years are leap years if they are exactly divisible by 400
So to evaluate if whether or not it is a leap year, we need to check whether:
- It is evenly divisible by 100
- If it is divisible by 100, then it should also be divisible by 400
- Except this, all other years evenly divisible by 4 are leap years
Expected Output:
- February 2016 has 29 days
6) Write a Java program to check whether a person is eligible to vote or not using the switch statement.
This Java program checks whether a person is eligible to vote based on their age.
- The program first prompts the user to enter their age and uses the ternary operator to check whether the age is greater than or equal to 18:
- If it is, set the value of your result variable to 1
- Otherwise, set it to 0
- The program should use a switch statement to print the corresponding message based on the value of your result variable:
- If the age is greater than or equal to 18, the program prints "Congratulations! You are eligible to vote!"
- Otherwise, the program prints "Sorry, you are not eligible to vote."
Lab 2: Basic Grade Calculator
The goal of this exercise is to program a simple Grade Calculator.
- First, prompt the user to enter their name (first and last as separate values) and their grade (as a double) Scanner to read in their response(s)
- Then, use conditional logic to determine their letter grade based on their numerical grade using the following:
- Finally, determine whether or not the student needs to retake the course. This is determined by if they have a grade other than A, B, or C.
- Write all output (not prompts!) to a plain text file: "[First]_[Last]_Grade.txt".
- Limit the student's numerical grade to two decimal places;i>
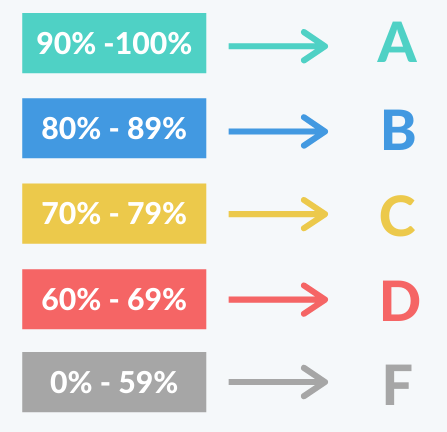
So if the prompts and input look like:
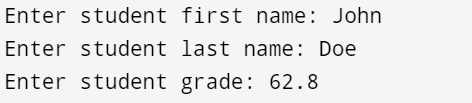
The output file: "John_Doe_Grade.txt" should look like:
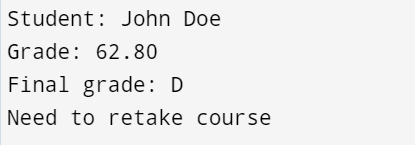
Submit both the .java and .txt file to EC Lab Submissions under Class Labs on Blackboard before next class. (This lab will count as part of your CodeLab grade)
Review
a) Write a switch statement that tests the value of the char variable response and performs the following actions:
- if response is y, the message Your request is being processed is printed
- if response is n, the message Thank you anyway for your consideration is printed
- if response is h, the message Sorry, no help is currently available is printed
- for any other value of response, the message Invalid entry; please try again is printed
(CodeLab: Chapter 5: Conditionals and logic > switch > #20623)
b) HTTP is the protocol that governs communications between web servers and web clients (i.e. browsers). Part of the protocol includes a status code returned by the server to tell the browser the status of its most recent page request.
Some of the codes and their meanings are listed below:
- 200, OK (fulfilled)
- 403, forbidden
- 404, not found
- 500, server error
Given an int variable status, write a switch statement that prints out, on a line by itself, the appropriate label from the above list based on status.
(CodeLab: Chapter 5: Conditionals and logic > switch > #20926)
1) Write a Java program that will read in a start number and an end number from a user. Then, using a while loop show all the values from the start to the end number, (inclusive).
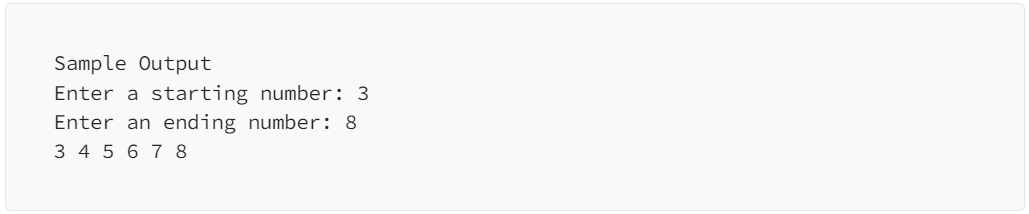
2) Write a program that will read in numbers from the user and sum them up. If the value entered by the user is 0 the program loop ends and the average of all the values entered is shown.
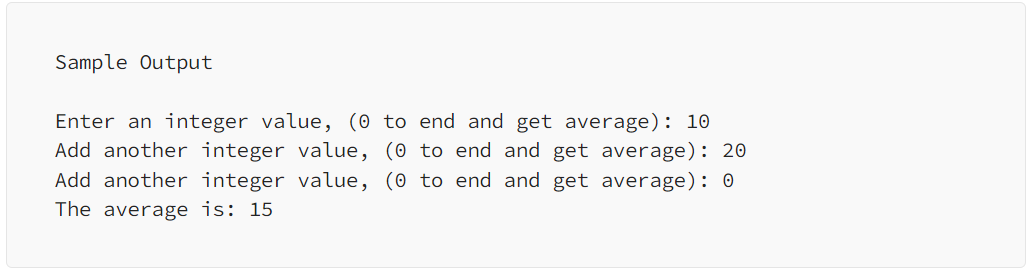
Review
a) Given int variables k and total that have already been declared, use a do/while loop to compute
the sum of the squares
of the first 50 counting numbers, and store this value in total.
Thus your code should put 1*1 + 2*2 + 3*3 +... + 49*49 + 50*50 into total.
Use no variables other than k
and total.
(CodeLab: Chapter 7: Loops > 7.6: The do-while loop > #20684)
b) For the following program:
public static void main(String [] args) {
int i = 10;
while (i > 1) {
System.out.println(i);
if (i % 2 == 0) {
i = i / 2;
} else {
i = i + 1;
}
}
}
What is the output of this program?
c) Write a Java program that prompts the user to enter the number whose factorial
they wish to compute and then calculates the factorial of that number using a while loop.
The factorial of any number n:
- is represented by n!
- and is equal to 1*2*3*....*(n-1)*n
And, 5! = 5 * 4 * 3 * 2 * 1 = 120
Note: The value of 0! is 1, according to the convention for an empty product.
Week 5
1) Write Java code for each the following tasks:
- Create a for loop that prints all even numbers between 1 and 20 (inclusive)
- Create a for loop that prints all odd numbers between 30 and 50 (inclusive)
- Create a for loop that prints the first 10 positive integers in descending order
- Create a for loop that calculates and prints the sum of the first 15 multiples of 5
Exam 1 Review
a) For the following program(s), identify the error (compiler, run-time, or logical) AND correct the following code so it will do what is explained:
1. The following section of code is supposed to initialize two numbers x and y and compute their sum.
int x = 5;
int y = "10";
int sum = x + y;
System.out.println("Sum: " + sum);
2. The following section of code is supposed to calculate the sum of the numbers 1 to n.
Scanner stdin = new Scanner(System.in);
int n = stdin.nextInt();
int sum;
for (int i = 1; i <= n; i++) {
sum = 0;
sum = sum + i;
}
System.out.println("sum = " + sum);
b) Make a simple program which will do the following tasks.
In your program, x and y should be two
variables that you declare and then
assign a value to -- either read the values in or use assignment statements.
1. Using the logical operator && (and), construct an if statement which will do the following:
Print "they are both positive" if the two variables are both greater than zero
For example, if x is 10 and y is 7.
Otherwise print the words: "they are not both positive"
(for example, if x is 10 and y is -6; or it might be that x is 0 and y is -3)
2. Using the logical operator || (or), construct an if statement which will do the following:
Print "at least one is positive" is either one (or both) of the two variables is greater than zero
For example, if x is -10 and y is 7; or it might be that x is 10 and y is 3 (in this case both are positive).
Otherwise print the words: "neither one is positive" (for example, if x is 0 and y is -6)
3. Using the logical operator ! (not), construct an if statement which will do the following:
Print "it is not positive" if one (you can pick either one to work with) of the two variables is not greater than zero -- for example, if x is -10.
Otherwise print the words: "it is positive" -- for example, if x is 6. (actually, this case is really saying it is not not positive)
c) Write a complete Java program (starting from import statement(s)) that reads in (from the keyboard) the following information about a book:
- Book ID (an integer)
- Title
- Author
- Genre (represented by an integer)
Based on the genre number of the book, the program should determine the genre and recommended age group for the book according to the table below:
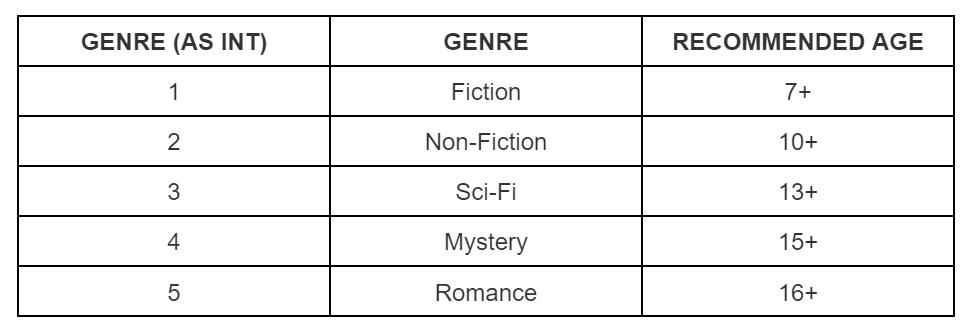
After determining the genre and recommended age group, the program should display the book's ID, title, author, genre, and recommended age group.
(when Book ID is 98765, title is "Superfudge", author is "Judy Blume", genre (int) is 1)
Sample output:
Book ID: 98765
Title: Superfudge
Author: Judy Blume
Genre: Fiction
Recommended Age Group: 7+
Keep reading in books (use a loop) until the user enters a Book ID number of -1.
Review
a) Write a for loop that prints in ascending order all the positive integers less than
or equal to 200 that are divisible by both 2 and 3, separated by spaces.
(CodeLab: Chapter 7: Loops > 7.5: The for statement > #20998)
Lab 3: Book Sorting Program
The goal of this exercise is to write a complete Java program that reads a list of books from a text file and categorizes them into separate genre files based on specific criteria
Write a complete Java program (starting from import statement(s)) that reads in from the text file "books.txt", the following information about a book:
- Book ID (an integer)
- Title
- Author
- Genre (represented by an integer)
And must create separate text files for each possible genre:
- "Fiction.txt"
- "NonFiction.txt"
- "SciFi.txt"
- "Mystery.txt"
- "Romance.txt"
For each book entry, determine the genre, based on the genre number of the book, according to the table below:
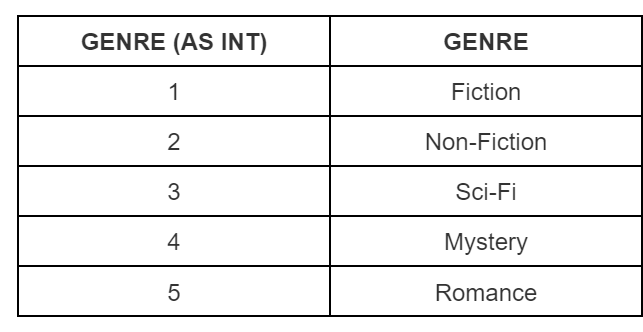
Write the book details in the appropriate genre file each on one line using the following format:
Book ID: [Book ID] Title: [Title] Author: [Author]
The program should loop until all entries in the "books.txt" file are processed.
After running your program, you should have the following output files ("Fiction.txt", "NonFiction.txt", "SciFi.txt", "Mystery.txt", "Romance.txt") and verify that each file contains the correct book entries
Submit the source code (.java file) and the FIVE output .txt files to EC Lab Submissions under Class Labs on Blackboard before next class. (This lab will count as part of your CodeLab grade)
Week 8
1) Write a Java program that reads in the user's first and last name and then prints out their initials.
Sample Input:
Enter name: John Doe
Sample Output:
Initials: J.D.
2) Consider the following String:
String hannah = "Did Hannah see bees? Hannah did.";
- What is the value displayed by the expression hannah.length()?
- What is the value returned by the method call hannah.charAt(12)?
- Write an expression that refers to the letter b in the String referred to by hannah.
3) Write a Java program to decode the following message:
Iqqf\"Lqd#\"[qw\"hkiwtgf\"kv\"qwv#
Each character in the original message has been shifted forward by 2.
To decode this message we will have to shift each character down by 2.
What does the message say after it is decoded?
4) What is printed by the following sections of code?
-
String source = "ID is 12-AB-1X "; int pos = source.indexOf('-'); int pos1 = source.indexOf(' ',pos+1); String str1 = source.substring(pos+1,pos1); System.out.println(str1);
-
source = "from Denver,CO."; int pos2 = source.lastIndexOf(','); int pos3 = source.lastIndexOf(' ',pos2); String str2 = source.substring(pos3+1,pos2); System.out.println(source.substring(pos3+1,pos2));
-
System.out.println(str1.compareTo(str2)>0);
5) A String variable sentence consists of words separated by single spaces.
Write the Java code needed to print the number of words that start with an upper case letter.
So for "The deed is done", your code would print 1 but for "The name is Bond, JAMES Bond", your code would print
4.
6) Write a Java Program that prompts the user to enter a String and counts the number of vowels
(a, e, i, o, u) in the String.
Print out the total count to the console.
Sample Input:
Enter a String: Hello World
Sample Output:
Number of vowels: 3
Review
a) Write a Java Program that prompts the user to enter a String and checks if the String is a
palindrome (reads the same forward and backward).
Print out a message to the console indicating whether the String is a palindrome or not.
Sample Input:
Enter a String: Wow
Sample Output:
String reversed: woW
This String is a palindrome.
b) Using the text file: Week9Emails.txt, write a
Java program that reads in the data (first name, last name, email address)
from the text file, extract the domain names from the email addresses,
and display a unique list of domain names on the console.
Sample Output:
Unique Domain Names:
gmail.com
yahoo.com
hotmail.com
outlook.com
aol.com
Week 9
1) Given the String variables name1 and name2,
write a fragment of code that assigns the larger of the two to the variable first
(assume that all three are already declared and that name1 and name2 have been assigned values).
(NOTE: "larger" here means alphabetically larger, not "longer".
Thus, "mouse" is larger than "elephant" because "mouse" comes later in the dictionary than "elephant"!)
(CodeLab: Chapter 9: Strings and things > 9.6: String comparison > #20602)
2) Consider this data sequence: "fish bird reptile reptile bird bird bird mammal fish".
Let's define a SINGLETON to be a data element that is not repeated immediately before or after itself in
the sequence.
So, here there are four SINGLETONs (the first appearance of "fish", the first appearance of "bird", "mammal",
and the second appearance of "fish").
Write some code that uses a loop to read a sequence of words,
terminated by the "xxxxx".
The code assigns to the variable n the number of SINGLETONs that were read.
For example: in the above data sequence it would assign 4 to n.
Assume that n has already been declared. but not initialized. Assume that there will be at least one word before
the terminating "xxxxx".
ASSUME the availability of a variable, stdin, that references a Scanner object associated with standard
input.
(CodeLab: Chapter 9: Strings and things > 9.6: String comparison > #21009)
Week 10
1) Write a void method called "printMessage()" that takes a String parameter and simply prints out the message to the console.
2) Create a void method called "calculateArea()" that takes two double parameters (length and width) and calculates the area of a rectangle using the formula: area = length * width. Print out the result to the console.
3) Write a Java method called "calcAverage()" that takes no arguments, prompts the user for
three numbers (double)
and then prints out the average of the three numbers with one decimal place.
Sample Input:
Input the first number: 25
Input the second number: 45
Input the third number: 65
Sample Output:
The average value is 45.0
4) Write a void method called "printPattern()" that takes an int parameter and
prints a pattern of asterisks to the console.
For example, if the parameter is 5, the output should be:
- *
- **
- ***
- ****
- *****
5) Write a complete Java program that has the following:
- A main method that should:
- prompt the user to enter values for the following variables: day, date, month, and year
- The variable day will contain the day of the week (like Friday)
- date will contain the day of the month (like the 13th)
- month should be from January to December
- year should be any sequence of 4 digits (like 2024)
- call the method printAmerican() to display the date in the American format
- call the method printEuropean() to display the date in the European format
- A method called printAmerican() that:
- takes the day, date, month and year as parameters
- displays them in standard American format, for example:
- Thursday, July 16, 2015
- returns nothing
- A method called printEuropean() that:
- takes the day, date, month and year as parameters
- displays them in standard European format, for example:
- Thursday 16 July 2015
- returns nothing
Week 11
1) What will be printed by the following:
public static void main(String [] args){
int a, b, c;
a = 1;
b = 8;
c = method(a, b);
System.out.printf("%d %d %d\n", a, b, c);
a = method(b, a);
System.out.printf("%d %d %d\n", a, b, c);
}
public static int method(int b, int a){
int d;
d = a * b - b * b;
return d + b;
}
2) Identify the following items in the program of Question 1:
- method header/signature
- method invocation
- method definition
3) Write a method biggest() that receives three int parameters. It will determine which value is the largest and return that value to the calling method.
4) Use the following formulas to write THREE overloaded calcPerimeter() methods, one for each shape. Each method should take the necessary parameters and return the perimeter as a double.
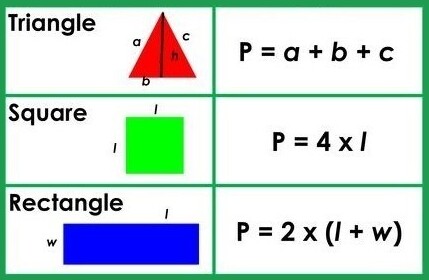
5) Write a complete Java program that prompts the user input to a String (can be an entire sentence)
and count the occurrences of each letter.
This program should use a for loop to loop through the
letters a - z (lowercase)
and call a method countOccurences() that takes two parameters: the String and a particular character
to search for and
return how many times that letter is found within the String.
The main method should then print
out this number to the console
for each individual letter in the String.
For example:
Sample Input:
Enter String: Hello World
Sample Output:
d appears 1 times
e appears 1 times
h appears 1 times
l appears 3 times
o appears 2 times
r appears 1 times
w appears 1 times
6) Write a method named isDivisible() that takes two double parameters,
n and m, and that returns
7) If you are given three sticks, you may or may not be able to
arrange them in a triangle. For example, if one of the sticks is 12 inches long
and the other two are one inch long, you will not be able to get the short sticks
to meet in the middle. For any three lengths, there is a simple test to see if it
is possible to form a triangle:
If any of the three lengths is greater than the sum of the other two,
you cannot form a triangle.
Write a method named isTriangle() that takes three integers as arguments
and returns either
8) What is the output of the following program?
public static void main(String [] args) {
boolean flag1 = isHoopy(202);
boolean flag2 = isFrabjuous(202);
System.out.println(flag1);
System.out.println(flag2);
if (flag1 && flag2) {
System.out.println("ping!");
}
if (flag1 || flag2) {
System.out.println("pong!");
}
}
public static boolean isHoopy(int x) {
boolean hoopyFlag;
if (x % 2 == 0) {
hoopyFlag = true;
} else {
hoopyFlag = false;
}
return hoopyFlag;
}
public static boolean isFrabjuous(int x) {
boolean frabjuousFlag;
if (x > 0) {
frabjuousFlag = true;
} else {
frabjuousFlag = false;
}
return frabjuousFlag;
}
9) Use the following formulas to write a Java program that has three methods:

- calculateVolumeOfSphere(double radius) that calculates and returns the volume of a sphere (in cubic units)
- calculateSurfaceAreaOfSphere(double radius) that calculates and returns the surface area of a sphere (in cubic units)
- isSphereLarge(double radius) that checks if a sphere with a given radius is considered large (volume
greater than 1000 cubic units)
and returns
true if it is,false otherwise.
The main method should print out the volume, surface area, and whether or not the sphere is large.
Lab 4: Processing Bowling Scores
The goal of this lab is to write a complete Java program to do the following:
The main program reads in a series of three bowling scores: score1, score2, and score3 from a file ("scores.txt"),
then calls a series of methods to process and print these scores: follow instructions found here
Submit the Java source code file (e.g., BowlingScores.java) and the program-generated output file (e.g.,
results.txt) file to EC Lab Submissions under Class Labs on Blackboard before next class. (This lab will count
as part of your CodeLab grade)
Week 12
1) What is the output of the following program?
public static void main(String [] args) {
int [] intArr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
for (int i = 0; i < intArr.length; i += 3) {
System.out.println("Value: " + intArr[i]);
}
}
2) What is the output of the following program?
public static void main(String [] args) {
int [] intArr = {1, 2, 3, 4, 5};
double [] dblArr = {0.5, 1, 1.5, 2, 2.5};
for (int i = 0; i < intArr.length; i++) {
dblArr[i] = dblArr[i] * intArr[i];
}
for (int i = 0; i < intArr.length; i++) {
System.out.println(intArr[i] + " : " + dblArr[i]);
}
}
3) Write a complete Java program that generates 20 random integers (between 1-100) and stores them in an array.
Then, use methods to count how many even, odd, or multiple of 5 appear in the set.
(Hint: write a method for each specific criteria)
Print the entire array to the console along with how many numbers fit each specified criteria.
Sample Output:
Generated random numbers:
{39, 87, 36, 57, 9, 29, 2, 39, 14, 35, 84, 74, 29, 69, 94, 89, 89, 54, 94, 42}
Even numbers count: 9
Odd numbers count: 11
Multiples of 5 count: 1
4) An array of ints, arr, has been declared and initialized. Write the statements needed to reverse the elements in the array.
So, if the elements were originally 5, 13, 4, 97 then after your code executes they would be 97, 4, 13, 5.
(CodeLab: Chapter 8: Arrays > 8.6: Array traversal > #20767)
Review
a) Given:
- an int variable k,
- an int array incompletes that has been declared and initialized,
- an int variable studentID that has been initialized, and
- an int variable numberOfIncompletes,
You may use only k, incompletes, studentID, and numberOfIncompletes.
Additional Notes: studentID and incompletes should not be modified
(CodeLab: Chapter 8: Arrays > 8.8: Traverse and count > #20716)
b) Show what is printed by the following program:
The data values to be used are as follows: 3 6 9 12 0 15 2 5 8 11
public static void main(String [] args){
String x;
int month;
Scanner sc = new Scanner (System.in);
do {
System.out.print("Enter any number: ");
month = sc.nextInt();
x = season(month);
System.out.println(month + " " + x);
} while (month != 8);
}
public static String season(int month){
String [] choices = {"winter", "spring", "summer", "fall", "error"};
if (month == 1 || month == 2 || month == 12) {
return choices[0];
} else if (month >= 3 && month <= 5) {
return choices[1];
} else if (month >= 6 && month <= 8) {
return choices[2];
} else if (month >= 9 && month <= 11) {
return choices[3];
} else {
return choices[4];
}
}
Week 13
1) Write a complete Java program that creates 2 parallel arrays and initialize them using initialization lists that represent the data below. Remember that the order of these arrays has to match so that you can use the same index and get corresponding values out.
- Countries: China, Egypt, France, Germany, India, Japan, Kenya, Mexico, United Kingdom, United States
- Capitals: Beijing, Cairo, Paris, Berlin, New Delhi, Tokyo, Nairobi, Mexico City, London, Washington D.C.
First, call a method, printMenu() to print a menu of the countries listed to screen and then prompt the user to enter a particular country.
Once you have read in the user's selected country, call a method, getCapital() to print out the country's corresponding capital.
This method should first call another method, findIndex() to find and return the specific index that the country is located at in the countries array.
- If findIndex() returns a -1, getCapital() should print out an error that the country is not found in the list.
- Otherwise, it should print out the corresponding capital (Hint: it should be located at the same index in the capitals array as the country in the countries array)
Review
a) Using the following array:

i) Show the process of performing a sequential (linear) search to find element: 23
ii) Then, show the process of performing a binary search to find element: 23
b) Consider an array of 10 integers that contains the following values in increasing sequence:
3 15 25 43 50 76 88 102 110 120
Assume you wish to find the location of the element with value 43.
- Show the sequence of numbers that you would encounter in this search if you used a sequential search.
- Show the sequence of numbers that you would encounter in this search if you used a binary search.
c) Given the array of numbers below, show all the steps as you perform a binary
search, looking for the key of 17:
int [] nums = {9, 15, 23, 67, 78, 85, 111, 117, 135, 225, 829, 1000};
Week 14
Lab 5: Count Zeroes
1) Write a complete Java program to do the following: follow instructions found here
Submit the Java source code file (e.g., CountZeros.java),
the two input files (The initial input file (initNums.txt)
and the secondary input file (e.g., moreNums.txt)) and
the program-generated output file (e.g., results.txt) file
to Lab Work under Class Labs on Blackboard before the next class.
(This lab will count as part of your CodeLab grade)
1) Given a random set of numbers, complete the method sortArr() which takes the array of integers and the size n as inputs and modifies the array in ascending sorted order. In the main method, print the numbers.
For example: if n = 4 and arr[] = {1, 5, 3, 2}
Output: {1, 2, 3, 5}
2) Write a complete Java program called, WordCounter, your program should:
- Prompt the user to enter a sentence
- Read in the sentence using the Scanner class
- Use the .split() method to split the sentence into an array of words based on spaces
- Print out the number of words in the sentence to the console.
For example: if the user enters "Hello World", your program should print out "Number of words: 2"
Sample exams: